As we know artificial intelligence is transforming many fields. Deep learning is one of the starts of the art model from a few decades. Convolutional neural network si one of the well know deep learning model. most of the time we train the model but we all think about that how we test the model in a real environment. In this project, you will learn how to make a multi-class image classification application using flask API.
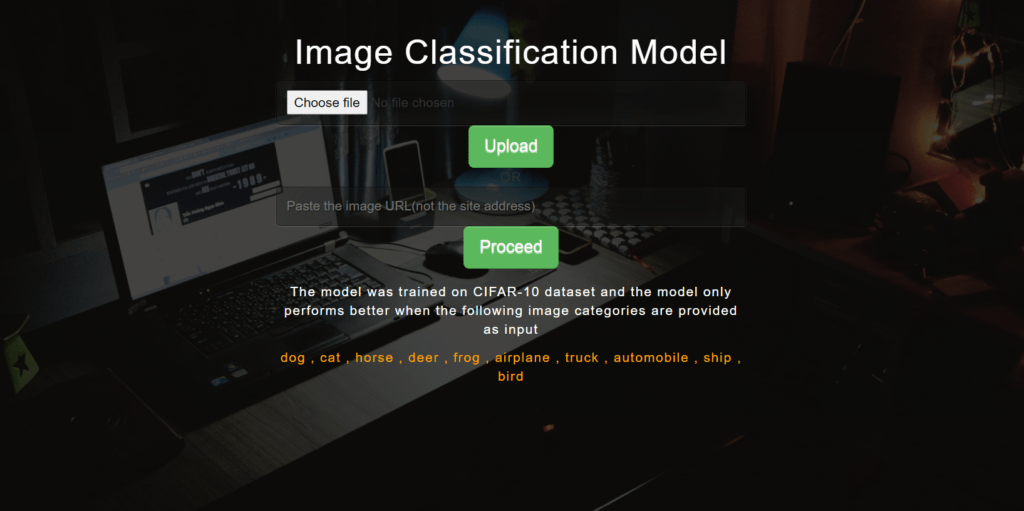
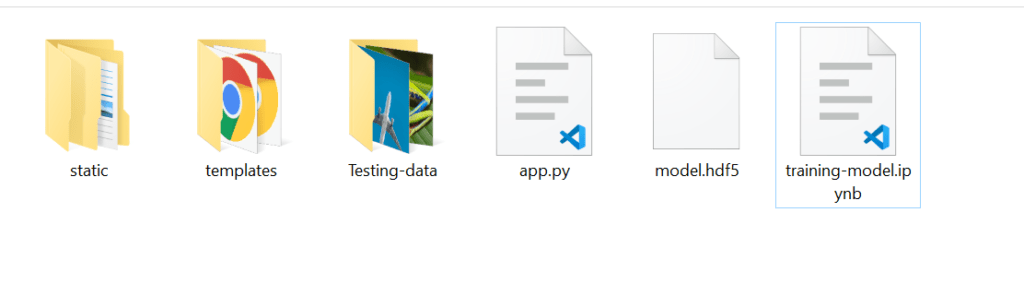
Before Runing this project make your have this liabriey install in your machine
Pip install keras, tensorflow, flask and more basic libraries if needed.
The proejct is mainly dvieded into two sets
- First Train the model
- Deploy the model in Flask App
CNN Model training code
import libraries
import tensorflow as tf import matplotlib.pyplot as plt from tensorflow.keras.preprocessing.image import ImageDataGenerator from tensorflow.keras.models import load_model from tensorflow.keras.models import Sequential from tensorflow.keras.preprocessing.image import ImageDataGenerator from tensorflow.keras.preprocessing.image import load_img , img_to_array from tensorflow.keras.layers import Dense , Dropout , Conv2D , MaxPooling2D, Flatten , BatchNormalization
Call Cifar-10 Dataset
(x_train , y_train) , (x_test , y_test) = tf.keras.datasets.cifar10.load_data()

check data and shape
print('x_train shape' , x_train.shape) print('y_train shape' , y_train.shape)

plt.imshow(x_train[1])
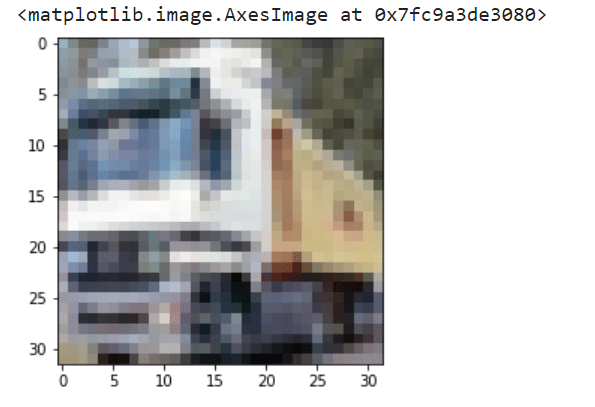
Data Normalization and Augmentation
def normalize(x): x = x.astype('float32') x = x/255.0 return x datagen = ImageDataGenerator( rotation_range=15, width_shift_range=0.1, height_shift_range=0.1, horizontal_flip=True, )
training test split
from sklearn.model_selection import train_test_split x_test, x_val, y_test, y_val = train_test_split(x_test, y_test, test_size = 0.5, random_state = 0)
x_train = normalize(x_train) x_test = normalize(x_test) x_val = normalize(x_val) y_train = tf.keras.utils.to_categorical(y_train , 10) y_test = tf.keras.utils.to_categorical(y_test , 10) y_val = tf.keras.utils.to_categorical(y_val , 10) datagen.fit(x_train)
Define Accuracy and loss graph and model fit function
def results(model): epoch = 100 r = model.fit(datagen.flow(x_train , y_train , batch_size = 32), epochs = epoch ,steps_per_epoch=len(x_train)/32, validation_data = (x_val , y_val) , verbose = 1) acc = model.evaluate(x_test , y_test) print("test set loss : " , acc[0]) print("test set accuracy :", acc[1]*100) epoch_range = range(1, epoch+1) plt.plot(epoch_range, r.history['accuracy']) plt.plot(epoch_range, r.history['val_accuracy']) plt.title('Classification Accuracy') plt.ylabel('Accuracy') plt.xlabel('Epoch') plt.legend(['Train', 'Val'], loc='lower right') plt.show() # Plot training & validation loss values plt.plot(epoch_range,r.history['loss']) plt.plot(epoch_range, r.history['val_loss']) plt.title('Model loss') plt.ylabel('Loss') plt.xlabel('Epoch') plt.legend(['Train', 'Val'], loc='lower right') plt.show()
convolutional neural network model
weight_decay = 1e-4 model = Sequential([ Conv2D(32, (3, 3), activation='relu', padding='same',kernel_regularizer=tf.keras.regularizers.l2(weight_decay), input_shape=(32, 32, 3)), BatchNormalization(), Conv2D(32, (3, 3), activation='relu',kernel_regularizer=tf.keras.regularizers.l2(weight_decay), padding='same'), BatchNormalization(), MaxPooling2D((2, 2)), Dropout(0.2), Conv2D(64, (3, 3), activation='relu',kernel_regularizer=tf.keras.regularizers.l2(weight_decay), padding='same'), BatchNormalization(), Conv2D(64, (3, 3), activation='relu',kernel_regularizer=tf.keras.regularizers.l2(weight_decay), padding='same'), BatchNormalization(), MaxPooling2D((2, 2)), Dropout(0.3), Conv2D(128, (3, 3), activation='relu',kernel_regularizer=tf.keras.regularizers.l2(weight_decay), padding='same'), BatchNormalization(), Conv2D(128, (3, 3), activation='relu',kernel_regularizer=tf.keras.regularizers.l2(weight_decay), padding='same'), BatchNormalization(), MaxPooling2D((2, 2)), Dropout(0.3), Flatten(), Dense(128, activation='relu'), Dense(10, activation='softmax') ]) opt = tf.keras.optimizers.SGD(lr=0.001, momentum=0.9) model.compile(optimizer=opt, loss='categorical_crossentropy', metrics=['accuracy']) results(model)
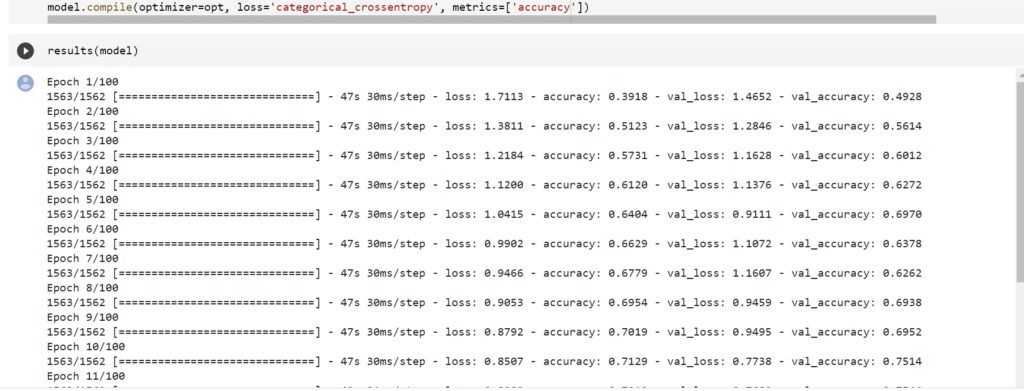
model save
model.save("model.hdf5")
Deploy model into Flask app
import os import uuid import flask import urllib from PIL import Image from tensorflow.keras.models import load_model from flask import Flask , render_template , request , send_file from tensorflow.keras.preprocessing.image import load_img , img_to_array app = Flask(__name__) BASE_DIR = os.path.dirname(os.path.abspath(__file__)) model = load_model(os.path.join(BASE_DIR , 'model.hdf5')) ALLOWED_EXT = set(['jpg' , 'jpeg' , 'png' , 'jfif']) def allowed_file(filename): return '.' in filename and \ filename.rsplit('.', 1)[1] in ALLOWED_EXT classes = ['airplane' ,'automobile', 'bird' , 'cat' , 'deer' ,'dog' ,'frog', 'horse' ,'ship' ,'truck'] def predict(filename , model): img = load_img(filename , target_size = (32 , 32)) img = img_to_array(img) img = img.reshape(1 , 32 ,32 ,3) img = img.astype('float32') img = img/255.0 result = model.predict(img) dict_result = {} for i in range(10): dict_result[result[0][i]] = classes[i] res = result[0] res.sort() res = res[::-1] prob = res[:3] prob_result = [] class_result = [] for i in range(3): prob_result.append((prob[i]*100).round(2)) class_result.append(dict_result[prob[i]]) return class_result , prob_result @app.route('/') def home(): return render_template("index.html") @app.route('/success' , methods = ['GET' , 'POST']) def success(): error = '' target_img = os.path.join(os.getcwd() , 'static/images') if request.method == 'POST': if(request.form): link = request.form.get('link') try : resource = urllib.request.urlopen(link) unique_filename = str(uuid.uuid4()) filename = unique_filename+".jpg" img_path = os.path.join(target_img , filename) output = open(img_path , "wb") output.write(resource.read()) output.close() img = filename class_result , prob_result = predict(img_path , model) predictions = { "class1":class_result[0], "class2":class_result[1], "class3":class_result[2], "prob1": prob_result[0], "prob2": prob_result[1], "prob3": prob_result[2], } except Exception as e : print(str(e)) error = 'This image from this site is not accesible or inappropriate input' if(len(error) == 0): return render_template('success.html' , img = img , predictions = predictions) else: return render_template('index.html' , error = error) elif (request.files): file = request.files['file'] if file and allowed_file(file.filename): file.save(os.path.join(target_img , file.filename)) img_path = os.path.join(target_img , file.filename) img = file.filename class_result , prob_result = predict(img_path , model) predictions = { "class1":class_result[0], "class2":class_result[1], "class3":class_result[2], "prob1": prob_result[0], "prob2": prob_result[1], "prob3": prob_result[2], } else: error = "Please upload images of jpg , jpeg and png extension only" if(len(error) == 0): return render_template('success.html' , img = img , predictions = predictions) else: return render_template('index.html' , error = error) else: return render_template('index.html') if __name__ == "__main__": app.run(debug = True)
Output
Complete project Download Code Link:
Thank you for the auspicious writeup. It in fact was a amusement account it.
Look advanced to far added agreeable from you!
By the way, how can we communicate?
What’s up, the whole thing is going fine here and ofcourse every one
is sharing facts, that’s in fact excellent, keep up writing.
Hello all, here every one is sharing these kinds of familiarity, thus
it’s good to read this website, and I used to pay a visit
this website everyday.
I would like to thank you for the efforts you have put in writing this blog.
I really hope to check out the same high-grade content from you in the future as well.
In fact, your creative writing abilities has motivated me to get
my very own site now 😉
I’m not sure exactly why but this web site is loading very slow for
me. Is anyone else having this problem or is it a issue on my end?
I’ll check back later on and see if the problem still exists.
Hi there this is somewhat of off topic but I was wondering if blogs use WYSIWYG editors or
if you have to manually code with HTML.
I’m starting a blog soon but have no coding experience so I wanted to get guidance from someone with experience.
Any help would be enormously appreciated!
Wow that was unusual. I just wrote an incredibly long comment but after I clicked submit my comment didn’t appear.
Grrrr… well I’m not writing all that over again. Anyhow, just wanted to say superb blog!
I’m gone to tell my little brother, that he should also pay a quick visit this blog on regular basis to take updated from most recent news.
Thank you for your articles. They’re very helpful to me. May I ask you a question?
I have to thank you for this article
I always find your articles very helpful. Thank you!
Your articles are very helpful to me. May I request more information?
Thanks for your help and for posting this. It’s been great.
I really appreciate your help
It would be nice to know more about that. Your articles have always been helpful to me. Thank you!
Thank you for writing so many excellent articles. May I request more information on the subject?
I want to thank you for your assistance and this post. It’s been great.
You’ve been a great aid to me. You’re welcome!
Someone essentially help to make seriously posts I would state. This is the very first time I frequented your web page and thus far? I surprised with the research you made to create this particular publish extraordinary. Wonderful job!
Hello there! Do you know if they make any plugins to assist
with SEO? I’m trying to get my blog to rank for some targeted keywords but I’m not seeing very good results.
If you know of any please share. Cheers!
My web site … nkcf.com