A Generative Adversarial Network (GAN) is a type of machine learning model that is composed of two parts: a generator and a discriminator. The generator model generates fake data, such as fake images, while the discriminator model attempts to classify the fake data as real or fake. The generator and discriminator models are trained together in a zero-sum game, where the generator tries to fool the discriminator and the discriminator tries to accurately classify the fake data.
GANs are often used for generating new data that is similar to a given training dataset. For example, you could use a GAN to generate new images that are similar to a dataset of real images. The generator model in a GAN learns to create fake data that is similar to the real data, while the discriminator model learns to accurately classify the fake data as real or fake. By training the two models together, the GAN is able to generate data that is similar to the real data but is not identical to any specific data point in the training dataset.
Overall, GANs are a powerful tool for generating new data and can be used for a variety of tasks, such as image generation, video generation, and text generation.
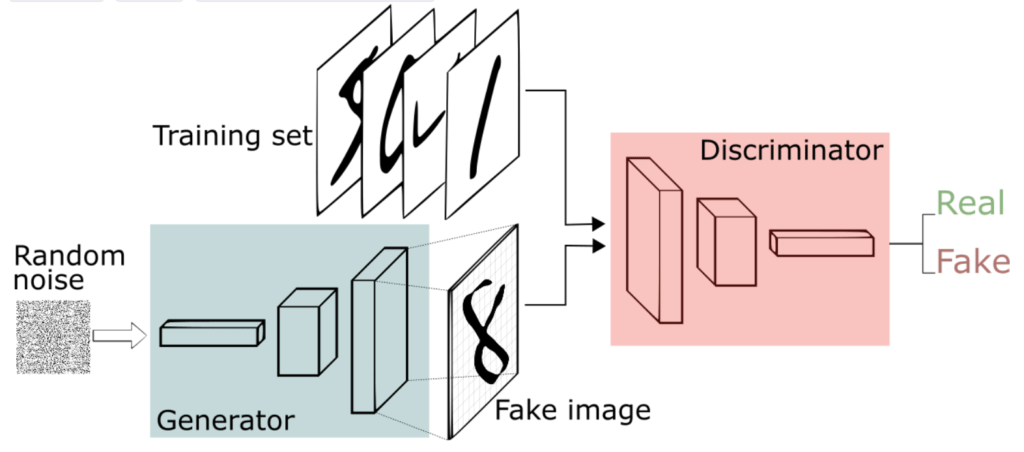
To write a code for a Generative Adversarial Network (GAN) model in Python, you can use the following steps:
- Import the necessary libraries, such as
numpy
andtensorflow
. - Define the generator and discriminator models. The generator model should take in random noise as input and generate fake images, while the discriminator model should take in both real and fake images and output a classification for each image (real or fake).
- Define the training loop for the GAN model. In each iteration, you will need to:
- Generate fake images using the generator model and random noise as input.
- Use the discriminator model to classify the real and fake images.
- Calculate the loss for the generator and discriminator models. The generator’s loss should be based on the discriminator’s classification of the fake images, while the discriminator’s loss should be based on its classification of both real and fake images.
- Use backpropagation to update the generator and discriminator models based on their losses.
- Use the trained generator model to generate new images using random noise as input.
Here is an example of what the code for a GAN model in Python might look like:
import numpy as np import tensorflow as tf # Define the generator model def generator(input_noise): # Define the model architecture here return generated_images # Define the discriminator model def discriminator(input_images): # Define the model architecture here return classification # Define the GAN model def gan(input_noise): # Generate fake images using the generator model fake_images = generator(input_noise) # Use the discriminator model to classify the fake images fake_classification = discriminator(fake_images) # Return the fake images and their classification return fake_images, fake_classification # Define the training loop for i in range(num_iterations): # Generate random noise noise = np.random.randn(batch_size, noise_dim) # Use the GAN model to generate fake images fake_images, fake_classification = gan(noise) # Use the discriminator model to classify real images real_images = # Get a batch of real images real_classification = discriminator(real_images) # Calculate the loss for the generator and discriminator models generator_loss = # Calculate the loss for the generator here discriminator_loss = # Calculate the loss for the discriminator here # Use backpropagation to update the generator and discriminator models generator_optimizer.apply_gradients(# Calculate the gradients for the generator here) discriminator_optimizer.apply_gradients(# Calculate the gradients for the discriminator here) # Use the trained generator model to generate new images noise = np.random.randn(batch_size, noise_dim) generated_images = generator(noise)
Note that this is just an example of how you could implement a GAN model in Python. There are many variations of GANs, so the exact architecture and training process may vary depending on your specific use case.
Generator architecture:
The architecture of a generator model in a GAN can vary depending on the specific use case and the type of data you are working with. In general, however, a generator model can be thought of as a deconvolutional neural network that takes in random noise as input and generates fake images as output.
Here is an example of a simple generator model architecture that you could use in a GAN:
def generator(input_noise): # Define the input layer inputs = tf.keras.layers.Input(shape=(noise_dim,)) # Use a dense layer to transform the noise input x = tf.keras.layers.Dense(256, activation='relu')(inputs) # Use a reshape layer to reshape the tensor into a 4D array x = tf.keras.layers.Reshape((8, 8, 8))(x) # Use a series of 3D transposed convolutional layers to upsample the tensor x = tf.keras.layers.Conv3DTranspose(128, (3, 3, 3), strides=(2, 2, 2), padding='same', activation='relu')(x) x = tf.keras.layers.Conv3DTranspose(64, (3, 3, 3), strides=(2, 2, 2), padding='same', activation='relu')(x) x = tf.keras.layers.Conv3DTranspose(32, (3, 3, 3), strides=(2, 2, 2), padding='same', activation='relu')(x) # Use a 3D convolutional layer to generate the final fake images generated_images = tf.keras.layers.Conv3D(1, (1, 1, 1), activation='sigmoid', padding='same')(x) # Return the generated images return generated_images
This generator model takes in noise with a shape of (batch_size, noise_dim)
and outputs fake images with a shape of (batch_size, height, width, depth, channels)
, where height
, width
, depth
, and channels
are determined by the architecture of the model. The model uses a series of 3D transposed convolutional layers to upsample the noise input and generate the fake images.
Note that this is just one example of a generator model architecture that you could use in a GAN. There are many other possible architectures that you could use, and the specific architecture that is best for your use case will depend on the type of data you are working with and the goals of your GAN model.
Discriminator architecture:
Similar to the generator model, the architecture of a discriminator model in a GAN can vary depending on the specific use case and the type of data you are working with. In general, however, a discriminator model can be thought of as a convolutional neural network that takes in real and fake images as input and outputs a classification for each image (real or fake).
Here is an example of a simple discriminator model architecture that you could use in a GAN:
def discriminator(input_images): # Define the input layer inputs = tf.keras.layers.Input(shape=(height, width, depth, channels)) # Use a series of 3D convolutional layers to downsample the image tensor x = tf.keras.layers.Conv3D(32, (3, 3, 3), strides=(2, 2, 2), padding='same', activation='relu')(inputs) x = tf.keras.layers.Conv3D(64, (3, 3, 3), strides=(2, 2, 2), padding='same', activation='relu')(x) x = tf.keras.layers.Conv3D(128, (3, 3, 3), strides=(2, 2, 2), padding='same', activation='relu')(x) # Use a reshape layer to flatten the tensor x = tf.keras.layers.Reshape((8, 8, 8))(x) # Use a dense layer to output the classification for each image classification = tf.keras.layers.Dense(1, activation='sigmoid')(x) # Return the classification for each image return classification
This discriminator model takes in images with a shape of (batch_size, height, width, depth, channels)
and outputs a classification for each image with a shape of (batch_size, 1)
. The model uses a series of 3D convolutional layers to downsample the input images and a dense layer to output the classification for each image.
Note that this is just one example of a discriminator model architecture that you could use in a GAN. There are many other possible architectures that you could use, and the specific architecture that is best for your use case will depend on the type of data you are working with and the goals of your GAN model.