Machine learning is a method of teaching computers to learn from data, without being explicitly programmed. It involves using algorithms to analyze and understand complex data, and to make predictions or decisions based on that data. There are many different types of machine learning, including supervised learning, unsupervised learning, and reinforcement learning. In supervised learning, the computer is given a labeled dataset, which means that the correct answers are provided for the algorithm to learn from. In unsupervised learning, the algorithm is given a dataset without labels, and it must find patterns and relationships within the data on its own. In reinforcement learning, the algorithm learns by trial and error, receiving rewards or punishments for its actions.
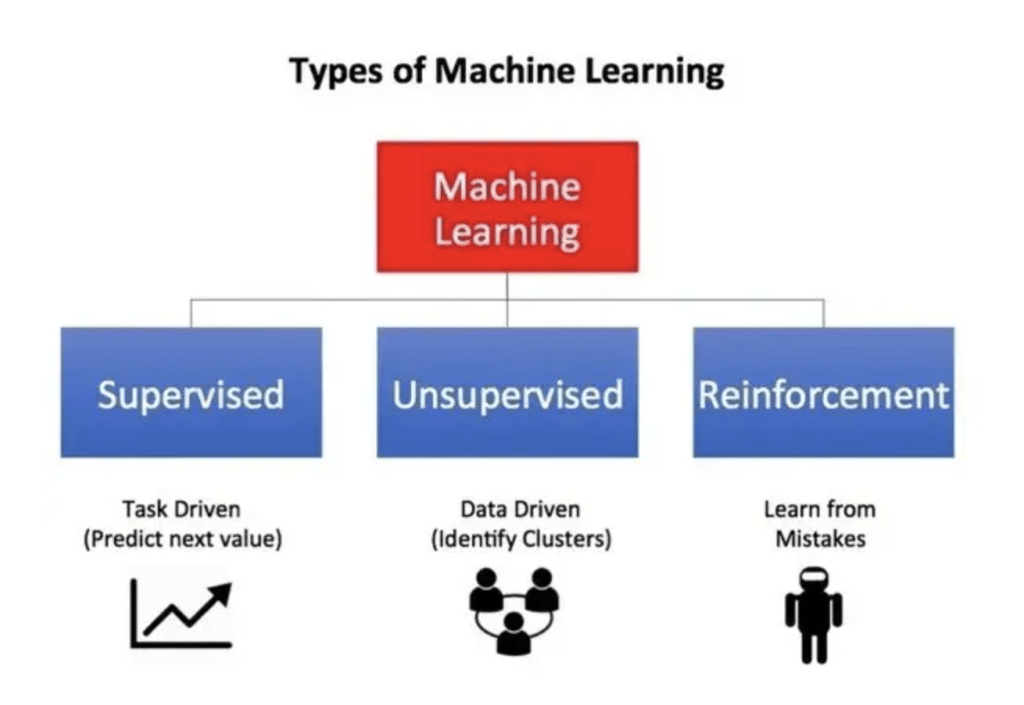
Supervised Learning example python
Here is an example of supervised learning in Python, using the scikit-learn library:
# Import the necessary libraries import numpy as np from sklearn import datasets from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Load the diabetes dataset diabetes = datasets.load_diabetes() X = diabetes.data y = diabetes.target # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3) # Train the model using a linear regression algorithm model = LinearRegression() model.fit(X_train, y_train) # Make predictions on the test data predictions = model.predict(X_test) # Evaluate the model's performance score = model.score(X_test, y_test) print(f"Model accuracy: {score}")
In this code, we use the scikit-learn library to load the diabetes dataset, which contains information about various medical factors that can affect a person’s diabetes progression. We then split the data into training and testing sets, and train a linear regression model on the training data. The model is then used to make predictions on the test data, and its performance is evaluated using the score
method. This is an example of supervised learning because the model is provided with labeled data, and it uses that data to learn the relationship between the input features and the target variable.
Unsupervised Learning example python
Here is an example of unsupervised learning in Python, using the scikit-learn library:
# Import the necessary libraries import numpy as np from sklearn import datasets from sklearn.cluster import KMeans # Load the Iris dataset iris = datasets.load_iris() X = iris.data # Use the K-Means algorithm to cluster the data into three groups kmeans = KMeans(n_clusters=3) kmeans.fit(X) # Print the cluster labels for each sample print(kmeans.labels_)
In this code, we use the scikit-learn library to load the Iris dataset, which contains information about different species of flowers. We then use the K-Means algorithm, which is a type of unsupervised learning algorithm, to cluster the data into three groups. The K-Means algorithm is given the data without labels, and it must find patterns and relationships within the data on its own. The labels_
attribute of the kmeans
object contains the cluster labels for each sample in the dataset. This is an example of unsupervised learning because the algorithm is not provided with labeled data, and it must find the clusters on its own.
Reinforcement Learning example python
Here is an example of reinforcement learning in Python, using the OpenAI Gym library:
# Import the necessary libraries import gym # Create a new environment env = gym.make("CartPole-v1") # Set the number of episodes to run num_episodes = 10 # Run the episodes for i in range(num_episodes): # Reset the environment at the start of each episode state = env.reset() # Run the episode until it's done while True: # Render the environment env.render() # Choose a random action action = env.action_space.sample() # Take the action and get the next state and reward next_state, reward, done, info = env.step(action) # Update the state state = next_state # If the episode is done, break out of the loop if done: break # Close the environment env.close()
In this code, we use the OpenAI Gym library to create a new environment, called “CartPole-v1”. This environment is a simple game where the goal is to balance a pole on a moving cart. At each step in the episode, the algorithm must choose an action (left or right) to take, and it receives a reward or punishment based on how well it is doing. This is an example of reinforcement learning because the algorithm learns through trial and error, receiving rewards or punishments for its actions.